Web Development with Python: Flask vs Django – Which One to Choose?
Sector: Technology
Author: Nisarg Mehta
Date Published: 01/28/2025
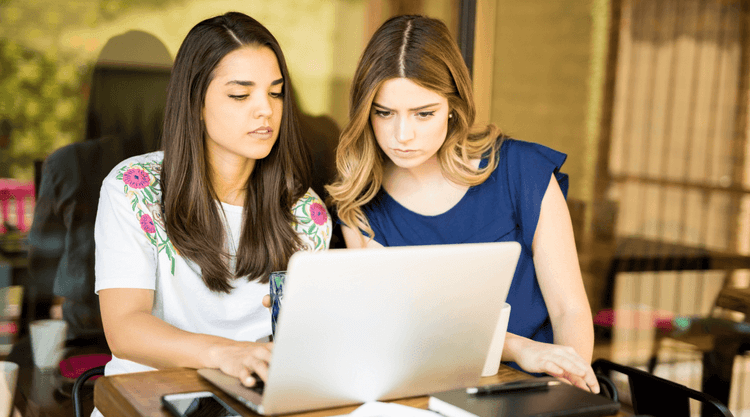
Contents
Python is the Swiss Army knife of programming languages when it comes to web development: it’s powerful, versatile, and pretty easy on the hands. Why? Python is not only easy to read, it is built for solving real-world problems, which many other languages are simply not.
Python is awesome; whether it is about handling massive data processing tasks, automating workflows integrating cutting-edge AI, etc. It’s also platform-independent so you can run your apps on just about any platform without worrying about compatibility.
And now, to be fair, even the best of tools need some right accessories to be used to the maximum. Enter Batman and Superman of Python web frameworks: Flask and Django. Now we know what each one is, so which one should you use for your next project? Let us dive into the nitty gritty to help you make the best decision.
Why Choose Python for Web Development?
Why do businesses (and the developers) love Python so much? It’s simple: Python is that friend who does it all if easy. Python’s clean syntax, large libraries, and a never-sleeping community make it a dream come true for even the most novice to the most experienced developer. Python is a language to build any sort of website, a simple portfolio website, or high-traffic eCommerce platform. Here’s a quick example:
1from textblob import TextBlob
2# Simple sentiment analysis
3text = "Python is an amazing programming language!"
4blob = TextBlob(text)
5print(f"Sentiment: {blob.sentiment.polarity}")
6
This example uses Python’s TextBlob library to determine the sentiment of a sentence in just a few lines of code. Can another language do it as concisely and readably? Many would struggle to match Python’s combination of simplicity, readability, and vast libraries tailored for such use cases. Python ensures even AI tasks are approachable for newcomers while remaining powerful enough for experts.
Python plays nicely with modern tech like AI, machine learning, and data science. It’s no wonder giants like Google, Instagram, and Spotify are all in on Python. If you’re looking to build web apps, Python’s web frameworks — Flask and Django — are your ultimate wingmen.
Overview of Flask
Flask’s one of its biggest strengths comes from being minimalist. This micro framework gives you total control to create your project in exactly the preferred way. Think about using a true painting surface rather than a kit with everything predetermined. You can pick and use the exact tools you need in Flask projects because it excludes unnecessary elements. It’s one of the best things about it, that it’s liberating, you’re back in control, and you have a lot of flexibility and freedom that you just won’t find in more opinionated frameworks.
Key Features of Flask:
- Lightweight and Flexible: Flask doesn’t come with too many bells and whistles. You only add what you need.
- Extensive Extensions: Need authentication? Database integration? Just pick the right extension and you’re good to go.
- Simple to Learn: Its simplicity makes Flask a fantastic choice for small projects or when you’re just starting out.
When to Use Flask:
- Building APIs or microservices.
- Developing small-to-medium web apps where speed and flexibility matter.
- When you want full control over your project’s architecture.
Overview of Django
Flask is a blank canvas, and Django is the preassembled LEGO set. A full-featured web framework with everything you need to build technology-exceeded web apps while needing to look elsewhere for missing pieces. Out of the box, Django includes an ORM, a built-in admin panel, and some solid security features. This framework is made for developers who need a powerful and time-saving framework. Django’s motto says it all: “In short, this is the web framework for perfectionists with deadlines.” Django is a perfectly great thing to build an eCommerce platform, content management system, or social app on if you’re thinking of building one of these. It’ll get you up and running quickly.
Key Features of Django:
- Batteries-Included: From an ORM (Object-Relational Mapping) to an admin panel, Django gives you everything out of the box.
- High-Level Security: Prevents common vulnerabilities like SQL injection and cross-site scripting.
- Scalable and Robust: Django can handle everything from small projects to enterprise-level web apps.
When to Use Django:
- Large, feature-rich applications like eCommerce sites or CMS platforms.
- Projects where security and scalability are top priorities.
- When you want to build fast without reinventing the wheel.
Pros and Cons of Flask and Django
Let’s get into a bit more detail and compare these two Python frameworks:
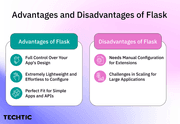
Flask Pros
- Super Lightweight and Easy to Set Up: Flask is so minimal that it’s a breeze to start. Take, for example, building a RESTful API to serve your data: it’s a couple of lines of code breeze. Flask is great because you’re not bogged down by all the unnecessary components, so it’s great for quick prototypes.
- Freedom to Design Your App: Unlike Django, Flask doesn’t force you into a predefined structure. For instance, if you prefer using SQLAlchemy over Django’s ORM, Flask allows you to integrate it seamlessly.
- Great for Smaller Apps and APIs: Imagine you’re building a lightweight microservice for processing user feedback. Flask’s modularity makes it an ideal fit, allowing you to keep things lean and performant.
Flask Cons
- Requires Manual Extension Setup: While Flask’s flexibility is its strength, it can also be its weakness. For example, if your project grows and you need user authentication, you’ll have to research, choose, and implement an extension manually, like Flask-Login or Flask-Security.
- Not Ideal for Large-Scale Projects: Managing a massive application with multiple components can become unwieldy without the built-in structure of Django. Imagine trying to scale an eCommerce app; Flask would require a lot of custom configurations to match Django’s robustness.
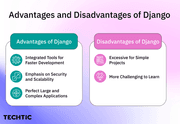
Django Pros
- Built-In Tools to Speed Up Development: Django admin panel can save you a lifetime of hassle in managing database records. For example, an example dashboard for creating, reading, updating, and deleting entries can be set up with minimal effort.
- Strong Focus on Security and Scalability: Features like SQL injection protection, CSRF tokens, and secure password hashing come baked into Django. A real-world use case is an online banking platform where security is paramount.
- Ideal for Large, Complex Applications: Imagine a content management system (CMS) like a blogging platform. As for the capabilities Django offers out of the box, like templating, ORM, user authentication, etc, it’s a great and straightforward choice.
Django Cons
- Can Feel Heavy for Simple Projects: If all you need is a basic API, Django’s extensive features might feel like overkill. Setting up a simple “to-do list” app, for example, can involve more effort than using Flask.
- Steeper Learning Curve: Mastering Django’s ecosystem—including its ORM, template system, and middleware—takes time. For beginners, this can be daunting compared to Flask’s simplicity.
Key Differences Between Flask and Django
Here’s where it gets interesting. Flask and Django are like apples and oranges — both fruit, but very different tastes.
Feature | Flask | Django |
Philosophy | Minimalistic and flexible | Batteries-included, all-in-one |
Learning Curve | Easy for beginners | Steeper but rewarding |
Performance | Faster for small apps | Optimized for large-scale apps |
Security | Relies on extensions | Built-in security features |
Community Support | Growing but smaller | Larger and more mature |
Flask vs Django: Which Python Framework is Right for You?
Deciding between Flask and Django is like deciding between two-star players on your team — both have their pros and cons, and the choice depends on the game you’re out to play. To assist you in making an informed decision, let’s break this down in greater detail.
A. Choose Flask If
The following are the situations when Flask is the right Python framework for you:
Small and Simple Projects
Flask is light enough for smaller projects or a prototype. For example, imagine you’re creating a basic weather app that gets data from an API and shows it on a webpage. If you are using Flask you can achieve this app without unnecessary components in less than 50 lines of code.
1from flask import Flask, jsonify
2import requests
3app = Flask(__name__)
4@app.route('/')
5def weather():
6 response = requests.get('https://api.weatherapi.com/v1/current.json?key=YOUR_KEY&q=London')
7 return jsonify(response.json())
8if __name__ == '__main__':
9 app.run(debug=True)
10
You Want Maximum Flexibility
Flask doesn’t impose a specific project structure. For instance, if you want to organize your files in a non-standard way, or if you prefer SQLAlchemy over an ORM like Django’s, Flask lets you do it your way without any complaints. This freedom makes it ideal for developers who value customization and control.
Building APIs and Microservices
Imagine working on a microservice architecture where each service performs a specific function, like user authentication or payment processing. Flask’s modularity and performance shine here. It’s commonly used for creating RESTful APIs with tools like Flask-RESTful.
B. Choose Django If
Here are the scenarios when Django is the right Python framework for you:
You’re Tackling a Large, Feature-Rich Project
Django is the framework required for building complex web applications like eCommerce platforms or social networks. If we take an online store for example. When working with Django you get user authentication, database migrations, and even a built-in admin panel to manage products and orders.
Security is a Top Priority
Developing d web app that deals with sensitive data? Django comes with baked-in security such as CSRF protection, SQL injection prevention, and secure password hashing. Another example would be a financial institution building an online banking platform that can surely reap the benefits of Django’s security-first approach.
You Need to Scale
With that, let’s assume that your startup is going to be launching a marketplace app that could potentially host thousands of users someday. This is well suited for Django’s scalability and robustness. On top of that, its ORM makes dealing with databases fairly easy and also allows you to move between databases such as PostgreSQL and MySQL with very little effort.
Flask vs. Django: A Comparison in Action
Imagine a scenario where two startups need web frameworks for their projects:
- Startup A wants to build a small task management tool for their internal team. Flask is a natural choice because of its simplicity, allowing rapid development without unnecessary features.
- Startup B is launching a social media platform with features like user profiles, multimedia uploads, and messaging. Django’s batteries-included approach ensures they can handle all these requirements efficiently.
Final Words
In the end, Flask and Django are both incredible tools in the Python ecosystem. Think of Flask as your minimalist, agile friend and Django as your all-in-one powerhouse. The best choice comes down to your project’s requirements and your personal development style.
If you want further assistance in picking the right Python framework for your web application, feel free to reach out to us. Our web software architects can help you identify the right framework for your web app.
Latest Tech Insights!
Join our newsletter for the latest updates, tips, and trends.